The code below is intended to be a basic reference to anybody trying to use VBA code for Powerpoint. It’s certainly not complete, but it is code I’ve used for various reasons. I will try to add to and improve this reference as I use more PowerPoint VBA code.
Referencing presentations, slides and shapes
'Set the pptPresentation variable to the active presentation Dim pptPresentation As Presentation Set pptPresentation = ActivePresentation
'Set the pptSlide variable to the active slide Dim pptSlide As Slide Set pptSlide = Application.ActiveWindow.View.Slide
'Set the pptSlide variable to a specific slide (the 4th slide) Dim pptSlide As Slide Set pptSlide = ActivePresentation.Slides(4)
'Set pptObject variable to the selected Object Dim pptObject As Object Set pptObject = ActiveWindow.Selection.ShapeRange
Count the number of slides
'Count the number of slides in the active presentation Debug.Print ActivePresentation.slides.Count
Get the slide index number of the current slide
'Get the slide number of the active slide Debug.Print Application.ActiveWindow.View.Slide.SlideIndex
Add slides
'Create a new blank slide in position 5 of the active presentation Dim pptSlide As Slide Set pptSlide = ActivePresentation.Slides.Add(5, ppLayoutBlank)
'Create a new blank slide at the end of the active presentation Dim pptSlide As Slide Set pptSlide = ActivePresentation.Slides.Add(ActivePresentation.Slides.Count + 1, ppLayoutBlank)
ppLayoutBlank is just one of a number of available layout options.
ppLayoutBlank ppLayoutChart ppLayoutChartAndText ppLayoutClipartAndText ppLayoutClipArtAndVerticalText ppLayoutFourObjects ppLayoutLargeObject ppLayoutMediaClipAndText ppLayoutMixed ppLayoutObject |
ppLayoutObjectAndText ppLayoutObjectOverText ppLayoutOrgchart ppLayoutTable ppLayoutText ppLayoutTextAndChart ppLayoutTextAndClipart ppLayoutTextAndMediaClip ppLayoutTextAndObject ppLayoutTextAndTwoObjects |
ppLayoutTextOverObject ppLayoutTitle ppLayoutTitleOnly ppLayoutTwoColumnText ppLayoutTwoObjectsAndText ppLayoutTwoObjectsOverText ppLayoutVerticalText ppLayoutVerticalTitleAndText ppLayoutVerticalTitleAndTextOverChart |
Delete Slides
'Delete a slide, slide 6 in this example
ActivePresentation.Slides(6).Delete
Move Slides
'Move the position of a slide, move slide 4 to become slide 2
ActivePresentation.Slides(4).MoveTo toPos:=2
Looping through all the slides of the active presentation
'Loop through each slide in the active presentation Dim pptSlide As Slide Dim pptShape As Shape For Each pptSlide In ActivePresentation.Slides 'Do something to each slide Next
Looping through all the shapes on the active slide
'Looping through all shapes on the active slide Dim pptSlide As Slide Dim pptShape As Shape Set pptSlide = Application.ActiveWindow.View.Slide For Each pptShape In pptSlide.Shapes 'Do something to each shape Next
Looping through all shapes on all slides
'Loop through all shapes on a all slides of active presentation Dim pptSlide As Slide Dim pptShape As Shape For Each pptSlide In ActivePresentation.Slides For Each pptShape In pptSlide.Shapes 'Do something to those shapes Next Next
Looping through all linked shapes on all slides
'Loop through all linked shapes on a all slides of active presentation Dim pptSlide As Slide Dim pptShape As Shape For Each pptSlide In ActivePresentation.Slides For Each pptShape In pptSlide.Shapes If pptShape.Type = msoLinkedPicture Or pptShape.Type = msoLinkedOLEObject Then 'Do something to those linked shapes End If Next Next
If you are applying a method, the other option is to apply the method to all shapes, even if they are not linked. You will need to handle the errors where that shape is not a linked shape.
'Loop through all linked shapes on a all slides of active presentation Dim pptSlide As Slide Dim pptShape As Shape For Each pptSlide In ActivePresentation.Slides For Each pptShape In pptSlide.Shapes On Error Resume Next 'Apply method to all shapes, even if some shapes will error On Error Goto 0 Next Next
Managing Links
Some of the methods related to links occur at the presentation level, whilst others occur at the shape level.
Presentation level methods
'Break all links
ActivePresentation.BreakLinks
'Update all links
ActivePresentation.UpdateLinks
Shape level methods
The code snippets in this section do not stand alone. They must be used where the pptShape has been set or defined through a loop.
'Break the link for a specific linked shape
pptShape.LinkFormat.BreakLink
'Update the link to a specific linked shape
pptShape.LinkFormat.UpdateLink
'Change the link type to Manual update
pptShape.LinkFormat.AutoUpdate = ppUpdateOptionManual
'Change the link type to automatic update
pptShape.LinkFormat.AutoUpdate = ppUpdateOptionAutomatic
'Change a link to go to a new source file
pptShape.LinkFormat.SourceFullName = "C:\Users\marks\ExcelBook.xlsx!Sheet1!R1C1:R20C20"
Shapes
'Create a shape and set to a variable Dim pptSlide As Slide Dim pptShape As Shape Set pptSlide = Application.ActiveWindow.View.Slide Set pptShape = pptSlide.Shapes.AddShape(Type:=msoShapeRectangle, _ Left:=50, Top:=50, Width:=50, Height:=50) 'Name the shape pptShape.Name = "myShape
'Display the sizes of the selected shape Dim msgText As String msgText = "Top: " & ActiveWindow.Selection.ShapeRange.Top & vbNewLine msgText = msgText & "Left: " & ActiveWindow.Selection.ShapeRange.Left & vbNewLine msgText = msgText & "Height: " & ActiveWindow.Selection.ShapeRange.Height & vbNewLine msgText = msgText & "Width: " & ActiveWindow.Selection.ShapeRange.Width & vbNewLine MsgBox msgText
Discover how you can automate your work with our Excel courses and tools.
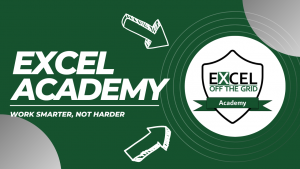
Excel Academy
The complete program for saving time by automating Excel.
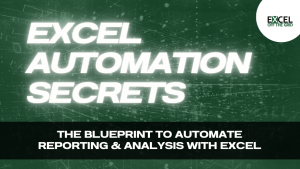
Excel Automation Secrets
Discover the 7-step framework for automating Excel.

Office Scripts: Automate Excel Everywhere
Start using Office Scripts and Power Automate to automate Excel in new ways.
Hi, I found this nice page on PowerPoint snippets, but could not find the VBA code to get a reference to an existing instance of PPT. It’s called CreateOrGetObject or GetOrCreateObject or something like that. If you ever update this page, that would be a useful snippet to include. I bookmarked this page in case I need some of these snippets one day. Thank you!
Thanks Kevin, that’s good feedback. I’ll add that in the next time I update it 🙂