At some point in your VBA life it will be necessary to display or re-order an array/list alphabetically (or reverse alphabetically). Unfortunately, VBA doesn’t have a function for sorting arrays automatically, but with a little bit of coding we can create a reusable function which would achieve something similar.
Setting up the example
This first VBA code is to set the scene. It creates an array, calls the function to sort the array and then outputs the sorted array. You only need this first piece of code if you are following it through as an example.
Sub RunTheSortMacro() Dim i As Long Dim myArray As Variant 'Set the array myArray = Array("p", "A", "G", 3, "l", "6", 10, "K", 7) 'myArray variable set to the result of SortArrayAtoZ function myArray = SortArrayAtoZ(myArray) 'Output the Array through a message box For i = LBound(myArray) To UBound(myArray) MsgBox myArray(i) Next i End Sub
Sort the array A-Z
I like to use a Function, rather than a Sub for sorting an array. The function is a reusable piece of code, it can form part of your ‘Core’ module, which you can copy into any VBA project. This function takes an array as its variable and outputs the same array sorted in alphabetical order.
Function SortArrayAtoZ(myArray As Variant) Dim i As Long Dim j As Long Dim Temp 'Sort the Array A-Z For i = LBound(myArray) To UBound(myArray) - 1 For j = i + 1 To UBound(myArray) If UCase(myArray(i)) > UCase(myArray(j)) Then Temp = myArray(j) myArray(j) = myArray(i) myArray(i) = Temp End If Next j Next i SortArrayAtoZ = myArray End Function
Sort the array Z-A
The reverse function to sort the array Z-A
Function SortArrayZtoA(myArray As Variant) Dim i As Long Dim j As Long Dim Temp 'Sort the Array Z-A For i = LBound(myArray) To UBound(myArray) - 1 For j = i + 1 To UBound(myArray) If UCase(myArray(i)) < UCase(myArray(j)) Then Temp = myArray(j) myArray(j) = myArray(i) myArray(i) = Temp End If Next j Next i SortArrayZtoA = myArray End Function
Discover how you can automate your work with our Excel courses and tools.
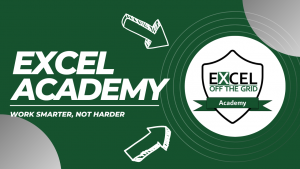
Excel Academy
The complete program for saving time by automating Excel.
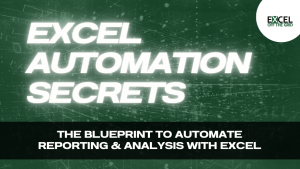
Excel Automation Secrets
Discover the 7-step framework for automating Excel.

Office Scripts: Automate Excel Everywhere
Start using Office Scripts and Power Automate to automate Excel in new ways.
The code look beautiful But you forgot to insert a Pic with sheet result
Thanks
For fast QuickSort sorting of large 1 or 2-dimensional arrays one can use WSTools from https://wizardsoft.nl/products/wstools .
ArraySort(Array.a, SortColumn = 0, Order = SORT_Ascending, Case = SORT_IgnoreCase)
Const SORT_Ascending = 0, SORT_Decending = 1
Const SORT_Case = 0, SORT_IgnoreCase = 1
Sorts Array by SortColumn with Order and Case sensitivity. Uses the QuickSort algorithm.
An alternative, especial for a 2d array, would be to write the array to a worksheet, apply the data menu sort function and read back the sorted array. For a 3+d array, we’re back to a modification of your method.
My version of the function:
Function SortArray(myArray As Variant, Optional SortOrder As Integer = 1) As Variant
Dim i As Long
Dim j As Long
Dim TempVal As Variant
Dim TempArr As Variant
On Error GoTo ErrHdl
TempArr = myArray
For i = LBound(TempArr) To UBound(TempArr) – 1
For j = i + 1 To UBound(TempArr)
If StrComp(TempArr(i), TempArr(j), vbBinaryCompare) = IIf(SortOrder = 1, 1, -1) Then
TempVal = TempArr(j)
TempArr(j) = TempArr(i)
TempArr(i) = TempVal
End If
Next j
Next i
SortArray = TempArr
Exit Function
ErrHdl:
SorrtArray = myArray
Following up/clarifying Deepak’s code, you will generally want to use the StrComp function in the If-statement instead of the “>” function. The “>” only works with single character strings as in the example. On the other hand, the StrComp is a native VBA command that compares entire strings alphabetically, character by character (and can be toggled between case specific and case independent).
For this example, the sort for longer strings would be:
If StrComp(myArray(i)), myArray(j)) = 1 Then
Note also how Deepak has the comparison set as a parameter so the same function can be used for AtoZ (=1) or ZtoA(=-1)
See this page for more on StrComp – https://docs.microsoft.com/en-us/office/vba/language/reference/user-interface-help/strcomp-function)