In the Windows Explorer it is possible to view a document’s properties. Right-click on the file and select Properties.
There is useful information in here, such as author, creation date, last saved date etc. The good news is, we can access this information using VBA with the BuiltinDocumentProperties Property.
Not all document properties are populated with data, as it depends on the file type. For example, Excel files do not have data about the number of slides property, but a PowerPoint file does. Yet, both Excel and PowerPoint files have a number of slides property, it’s just not used in all circumstances.
The basic VBA code
'Finding the author of the ActiveWorkbook Debug.Print ActiveWorkbook.BuiltinDocumentProperties("Author") 'Finding the creation date of another workbook Dim Wb As Workbook Set Wb = Workbooks("myFileTest.xlsx") Debug.Print Wb.BuiltinDocumentProperties("Creation date")
Working with closed files
By using the BuiltinDocumentProperties there is no way to read the properties from a closed file. Therefore it is necessary to open the file, read the properties, then close the file.
'Find the Last saved time of a currently closed file Dim Wb As Workbook Set Wb = Workbooks.Open("C:\Users\marks\Documents\myFileTest.xlsx") Debug.Print Wb.BuiltinDocumentProperties("Last save time") Wb.Close
Note: Take a look in the comments section below as there appears to be a way to read and change properties on a closed file. But that is outside the scope of this post.
Catching errors
Referencing a property which does not have any information will throw and error.
It is possible to catch the errors so that you know the value is blank:
'Catching errors when there is no value Dim fileProperty As Variant fileProperty = "Number of slides" On Error Resume Next Debug.Print ActiveWorkbook.BuiltinDocumentProperties(fileProperty) If Err.Number <> 0 Then Debug.Print fileProperty & " is blank" End If On Error GoTo 0
All the available properties
The available properties are:
Title Subject Author Keywords Comments Template Last author Revision number Application name Last print date Creation date Last save time |
Total editing time Number of pages Number of words Number of characters Security Category Format Manager Company Number of bytes Number of lines Number of paragraphs |
Number of slides Number of notes Number of hidden Slides Number of multimedia clips Hyperlink base Number of characters (with spaces) Content type Content status Language Document version |
It is also possible to loop through and list all of the available properties:
'List all the file properties available Dim fileProperty As Variant For Each fileProperty In ActiveWorkbook.BuiltinDocumentProperties Debug.Print fileProperty.Name Next fileProperty
Discover how you can automate your work with our Excel courses and tools.
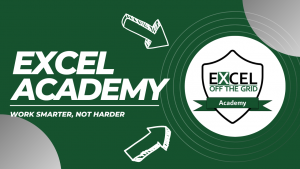
Excel Academy
The complete program for saving time by automating Excel.
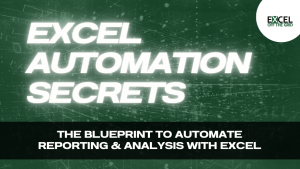
Excel Automation Secrets
Discover the 7-step framework for automating Excel.

Office Scripts: Automate Excel Everywhere
Start using Office Scripts and Power Automate to automate Excel in new ways.
What about DSOFile.dll (DSO File properties reader). Does that no longer work?
Hi Roy,
Thanks for the question. I must admit that I’ve not tried. It’s outside my current knowledge and skill level. It is something I will look into, as it’s always good to learn.
I will add a comment to the post to clarify that it’s only referring to the basic BuiltinDocumentProperties properties.